Build a quick inference CLI for your machine learning model
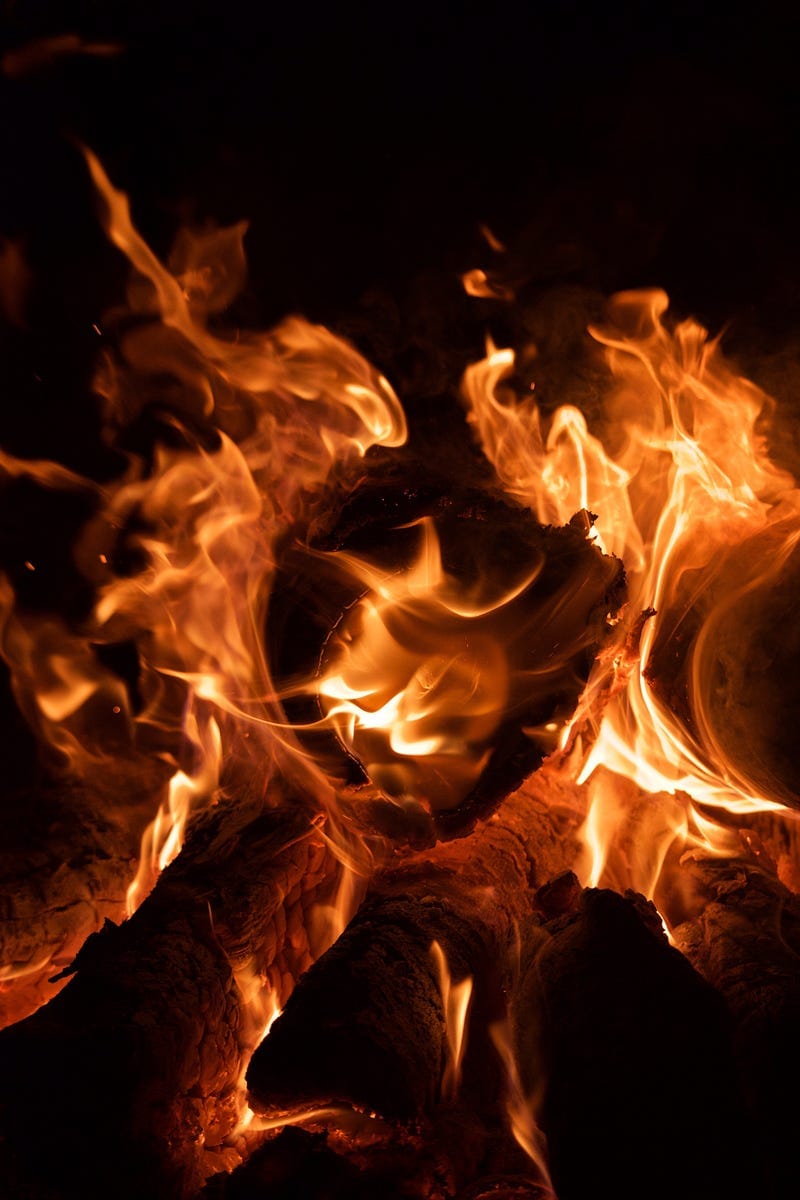
Convert your machine learning python script to a quick CLI tool in a modern python style using fire and poetry
Here we are going to quickly look at the python-fire package from google to bootstrap your python script into a CLI tool.
“Python Fire is a library for automatically generating command-line interfaces (CLIs) from absolutely any Python object.”- Google
You can find all the quick examples from this Github repo: https://github.com/google/python-fire
but here we will look into a few extended use cases of fire.
Quick Usage
Create a python file and start with a simple script:# myscript.pyfrom typing import Unionfrom somewhere import modelimport fire
def predict(height: Union[int, float], weight: Union[int, float]) -> Union[int, float]:
return model.predict([height, weight])
if __name__ == "__main__":
fire.Fire(add)
Now, if you can run this file as a CLI using -m
flag and passing 2 arguments in python.python -m myscript 155 60
Output
MALE
that was pretty quick and easy.
Class example
You can also create a CLI from the python class. Here is an example:import fire
from somewhere import model
class Predict:
@staticmethod
def gender(weight, model):
return model.predict_gender(weight, model)
@staticmethod
def age(weight, b):
return model.predict_age(weight, model)
@staticmethod
def BMI(weight, b):
return a / b
@staticmethod
def shoe_size(weight, height):
return model.get_shoe_size(weight, height)
if __name__ == "__main__":
fire.Fire(Predict)
We now have all those different methods we want in our calculator. We can run this using the following command:
- For age prediction:python -m myscript age 155 60Output
30
2. for shoe size prediction:
python -m myscript
Output
44
And so on….
Poetry Integration
Poetry is a package manager for python similar to npm
for NodeJS.
Poetry deserves a separate story, but if you want to learn more from the docs, here it is: https://python-poetry.org/.
We can integrate fire CLI with poetry for easy scripting. Here is a way to do that:
- Create a script and a runner file.
- In the runner file import the script that you want to run and put the name-main guard.# runner.py
from myscript import Predictdef main():
fire.Fire(Predict)
if __name__ == "__main__":
main()
3. Add an entry point in your `pyproject.toml` file below your dependencies.[tool.poetry.scripts]
predict = "runner:main"
4. Install your packages
poetry install
5. Now you can run your script simply using this command:predict age 155 60
Output
30
As easy as that 😀.

Comments ()